일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 정보처리기사
- Jackson
- 코드숨
- jsp
- java
- 주간회고
- 성적프로그램
- Python
- 미니프로젝트
- algorithms
- 필기
- 항해99
- 알고리즘
- 함수형 코딩
- 서평
- If
- 2020년 일정
- 2020년 정보처리기사 4회
- 2020년 제4회 정보처리기사 필기 문제 분석
- sqldeveloper
- Real MySQL
- LeetCode
- post
- hackerrank
- Til
- 뇌정리
- 스터디
- 책리뷰
- git
- 회고
- Today
- Total
조컴퓨터
200909 OOP 14 - Java 객체지향 프로그래밍 (Ⅱ) 본문
문제1) 상품 구매 프로그램 만들기
반복해서 보기
package oop0909;
import java.util.*;
//1)
class Product extends Object {
public int price; //상품가격
public int bonusPoint; //마일리지
public Product() {}
public Product(int price) {
this.price = price;
this.bonusPoint=(int)(price*0.1); //상품가격 10%를 보너스 점수 책정
}
}//class end
//2)
class SmartTV extends Product {
public SmartTV() {
super(100); //가격 price=100, bonusPoint=10
}
//상품명 등록
@Override
public String toString() {
return "스마트TV";
}
}//class end
class Notebook extends Product {
public Notebook() {
super(200); //가격 price=200, bonusPoint=20
}
//상품명 등록
@Override
public String toString() {
return "노트북";
}
}//class end
class Handphone extends Product {
public Handphone() {
super(150); //가격 price=150, bonusPoint=15
}
@Override
public String toString() {
return "핸드폰";
}
}//class end
//3) 상품을 구매하는 사람
class Buyer{
private int money=1000; //나의 총재산
private int mileage=0; //나의 마일리지 점수
//상품을 구매했을 때 어떤 상품을 구매했는지 저장(나의 구매목록)
private Product[] items=new Product[10];
//배열은 메모리를 정적으로 사용한다.
//할당해 놓은 만큼만 사용 그러나 우리는 메모리를 동적으로 사용한다. Vector
private int idx=0; //idx는 위의 인덱스에 접근하기 위해 설정해 놓은 값
public Buyer() {}
/* method overloading 함수 중복 정의
public void buy(SmartTV s) {}
public void buy(Notebook n) {}
public void buy(Handphone h) {}
*/
//SmartTV, Notebook, Handphone의 공통점은 부모가 Product이다.
public void buy(Product p) {
if(this.money<p.price) {
System.out.println("잔액이 부족합니다");
return;
}//if end
items[idx]=p; //구매한 상품 저장
idx=idx+1;
//나의 재산은 감소
this.money=this.money-p.price;
//나의 마일리지 점수
this.mileage=this.mileage+p.bonusPoint;
}//buy() end
public void disp() {
//구매 상품 목록
String list="";
//사용한 총금액
int hap=0;
for(int i=0; i<items.length; i++) {
if(items[i]==null) { //구매한 상품이 있을 때까지만
//없으면 반복문 빠져나감
break;
}//if end
hap=hap+items[i].price;
list=list+items[i].toString()+" ";
}//for end
System.out.println("구매 상품 목록: " + list);
System.out.println("사용 금액: " + hap);
System.out.println("남은 재산: " + this.money);
System.out.println("마일리지: " + this.mileage);
}//disp() end
}//class end
public class Test01_Buyer {
public static void main(String[] args) {
//상품 구매 및 반품 프로그램
//4) 상품 생성
SmartTV tv=new SmartTV();
Notebook note=new Notebook();
Handphone phone=new Handphone();
//5) 상품 구매
Buyer kim=new Buyer();
kim.buy(tv); //100
kim.buy(note); //200
kim.buy(phone); //150
kim.buy(phone);
kim.buy(phone);
kim.buy(phone);
kim.buy(phone);
//6) 출력
kim.disp();
}//main() end
}//class end
1)
- 상품에 대한 정보가 올라가야 하기 때문에 Product 클래스를 선언하고, 멤버 변수로 public int형의 상품가격, 상품을 구입하면 주는 마일리지를 선언한다.
- Product 클래스의 생성자 메소드로 초기화한다.
- 메소드 오버로드한 Product 클래스에 int price를 매개 변수로 받는 메소드를 선언하고 이때 보너스 포인트값은 상품가격의 0.1으로 연산하는 기능을 준다.
2)
- Product 클래스를 상속받는 SmartTV, Notebook, Handphone을 선언한다.
- 각각의 클래스 내부에 super 메소드로 부모의 상품가격을 물려 받는 생성자 메소드와 상품명을 리턴하는 toString 메소드를 만든다.
3)
- Buyer 클래스를 선언한다.
- private 제한자로 가지고 있는 나의 총재산과 마일리지를 변수로 선언한다.
- 구매할 상품을 저장할 Product[ ] 공간을 10개 마련한다.
- Buyer 클래스의 생성자 메소드로 초기화한다.
- Product p를 매개 변수로 하는 buy 메소드를 선언하고 가지고 있는 돈이 부족할 때 "잔액이 부족합니다"를 리턴할 수 있는 조건문을 만든다.
- idx++하여 Product[ ] 배열에 해당값(상품가격)을 저장한다.
- 출력을 하기 위해 disp 메소드를 선언한다.
- String list=" "을 선언하여 list에는 구매할 상품명을 저장한다.
- int hap=0을 선언하여 구매할 상품의 총 금액을 저장한다.
- 상품을 저장해 둔 Product[ ]의 갯수를 토대로 Product[ ]가 존재하지 않으면(null) 작업을 중지하고 존재하면 해당 상품의 가격을 더해 hap 변수 안에 저장한다.
- 구매 상품 목록, 사용 금액, 남은 재산, 마일리지를 출력한다.
4)
- 상품 구매를 하려면 메인 메소드에 상품이 올려져 있어야 한다. 이를 new 연산자를 통해 생성한다.
5)
- main 메소드 안에 Buyer 클래스의 kim이라는 new 연산자로 Buyer 객체를 선언한다.
- 구매자 kim에 buy 메소드 인자로 tv, note, phone, phone, phone, phone, phone을 입력해 준다.
6)
- 구매자 kim이 disp( ) 메소드로 출력하면 연산된 결과들이 출력된다.
문제2) 상품 구매 후 반품 프로그램 만들기
package oop0909;
import java.util.*;
class Product extends Object {
public int price; //상품가격
public int bonusPoint; //마일리지
public Product() {}
public Product(int price) {
this.price = price;
this.bonusPoint=(int)(price*0.1); //상품가격 10%를 보너스 점수 책정
}
}//class end
class SmartTV extends Product {
public SmartTV() {
super(100); //가격 price=100, bonusPoint=10
}
//상품명 등록
@Override
public String toString() {
return "스마트TV";
}
}//class end
class Notebook extends Product {
public Notebook() {
super(200); //가격 price=200, bonusPoint=20
}
//상품명 등록
@Override
public String toString() {
return "노트북";
}
}//class end
class Handphone extends Product {
public Handphone() {
super(150); //가격 price=150, bonusPoint=15
}
@Override
public String toString() {
return "핸드폰";
}
}//class end
//상품을 구매하는 사람
class Buyer{
private int money=1000; //나의 총재산
private int mileage=0; //나의 마일리지 점수
private int myProduct=0;
//상품을 구매했을 때 어떤 상품을 구매했는지 저장(나의 구매목록)
Vector<Product> items=new Vector<Product>();
//private int idx=0;
public Buyer() {}
/* method overloading 함수 중복 정의
public void buy(SmartTV s) {}
public void buy(Notebook n) {}
public void buy(Handphone h) {}
*/
//SmartTV, Notebook, Handphone의 공통점은 부모가 Product이다.
public void buy(Product p) {
if(this.money<p.price) {
System.out.println("잔액이 부족합니다");
return;
}//if end
items.add(p);
//나의 재산은 감소
this.money=this.money-p.price;
//나의 마일리지 점수
this.mileage=this.mileage+p.bonusPoint;
}//buy() end
public void disp() {
//구매 상품 목록
String list="";
//사용한 총금액
int hap=0;
for(int i=0; i<items.size(); i++) {
if(items.isEmpty()) { //구매한 상품이 있을 때까지만
//없으면 반복문 빠져나감
System.out.println("구매한 상품 없음");
return;
}//if end
Product p=items.get(i); //Product p에 get한 items의 0부터 ~~~ 까지의 값을 대입
hap=hap+p.price;
list=list+p.toString()+" ";
}//for end
System.out.println("구매 상품 목록 : " + list);
System.out.println("사용 금액 : " + hap);
System.out.println("남은 재산 : " + this.money);
System.out.println("마일리지 : " + this.mileage);
}//disp() end
public void refund(Product p) {
if(items.remove(p)) {
System.out.println(p.toString() + "이 반품 되었습니다");
this.money=this.money+p.price;
this.mileage=this.mileage-p.bonusPoint;
}else {
System.out.println("구매 내역에 상품이 없습니다");
}//if end
}//refund() end
}//class end
public class Test02_Buyer {
public static void main(String[] args) {
//상품 구매 및 반품 프로그램
//상품 생성
SmartTV tv=new SmartTV();
Notebook note=new Notebook();
Handphone phone=new Handphone();
//상품 구매
Buyer kim=new Buyer();
kim.buy(tv); //100
kim.buy(note); //200
kim.buy(phone); //150
//출력
kim.disp();
//상품을 중복 구매하지 않았다는 전제하에 반품
System.out.println("-------반품");
kim.refund(note);
System.out.println("-------결과");
kim.disp();
}//main() end
}//class end
상품 저장의 형식이 바뀌었다.
private Product[] items=new Product[10]; → Vector<Product> items=new Vector<Product>();
배열 객체는 메모리를 정적으로 사용하지만, 벡터 객체는 메모리를 동적으로 사용한다.
물건을 한 번에 10개 이상 구매하는 경우는 첫 번째 저장 형식으로는 진행할 수 없다.
이 경우에는 동적으로 형식을 저장할 수 있는 벡터 객체가 좀 더 적합하다.
환불 기능이 추가되었다.
- Buyer 클래스 내부에 Product p를 매개 변수로 하는 refund 메소드를 선언한다.
- Vector<Product>에 있는 상품 p를 제거하고 해당 p 상품명의 반품이 완료 되었음을 알린다.
- 이때 해당 상품 p의 상품가격만큼 구매자 kim의 총재산에 더해주고, 해당 상품 p를 구매했을 때 생성된 마일리지를 구매자 kim의 마일리지에서 감한다.
- Vector<Product>에 상품 p가 존재하지 않는다면 "구매 내역에 상품이 없습니다"를 출력하고 마친다.
출력 서식 Print Format
\n 줄바꿈
\t 탭
\r
\b
예제1) 출력 서식
System.out.println("\\"); //\
System.out.println("\""); //"
System.out.println("\'"); //'
System.out.print("사과\n\n\n");
System.out.print("수\n\n박");
System.out.print("\n참\t\t외\n");
/*
사과
수
박
참 외
*/
사과\n\n\n → '사과' 작성 후 줄바꿈 3번
수\n\n박 → '수' 작성 후 줄바꿈 2번 '박' 작성
\n참\t\t외\n → 줄바꿈 1번 '참' 작성 탭 2번 '외' 작성 줄바꿈 1번
%d 10진 정수형
%f 실수형
%c 문자형
%s 문자열형
예제2) 정수형, 실수형, 문자형, 문자열형의 출력 서식
System.out.printf("a:%d b:%d c:%d\n", 3,5,7); //a:3 b:5 c:7
System.out.printf("#%5d#\n", 123); //# 123#
System.out.printf("#%-5d#\n", 123); //#123 #
System.out.printf("#%05d#\n", 123); //#00123#
예제3) 여러 가지 출력 서식 - ①
System.out.printf("x:%f y:%f z:%f\n", 1.2, 3.4, 5.6); //x:1.200000 y:3.400000 z:5.600000
System.out.printf("#%6.2f#\n", 7.8); //# 7.80#
System.out.printf("#%-6.2f#\n", 7.8); //#7.80 #
System.out.printf("#%.2f#\n", 7.8); //#7.80#
System.out.println(10/3); //3
System.out.println(10/3.0); //3.3333333333333335
System.out.printf("#%.2f#\n", 10/3.0); //#3.33#
System.out.println(String.format("#%.4f#\n", 10/3.0)); //#3.3333# String
예제4) 여러 가지 출력 서식 - ②
System.out.printf("%c %c %c \n", 'S', 'K', 'Y'); //S K Y
System.out.printf("#%5c#\n", 'R'); //# R#
System.out.printf("#%-5c#\n", 'r'); //#r #
예제5) 여러 가지 출력 서식 - ③
System.out.printf("%s %s %s \n", "Year", "Month", "Date"); //Year Month Date
System.out.printf("#%8s#\n", "HAPPY"); //# HAPPY#
System.out.printf("#%-8s#\n", "HAPPY"); //#HAPPY #
File 클래스
- java.io 패키지는 기존의 파일이나 폴더에 대한 제어를 하는 데 사용하는 File 클래스를 제공한다.
- 첨부형 게시판, 이메일에서 전송한 파일이 어떠한 파일인지 확인하려면 File 클래스를 사용해야 한다.
- 파일과 관련한 정보를 알 수 있다.
예) 파일명, 확장명, 파일 타입, 파일 크기, ~
try {
//예외 발생이 예상되는 코드 작성
//경로명+파일명
//String pathname="C:\\java0812\\setup\\python-3.8.5.exe";
//경로명으로 /기호도 사용 가능하다
String pathname="C:/java0812/setup/python-3.8.6.exe";
//File 클래스에 담기
File file=new File(pathname);
if(file.exists()) { //파일이 존재하는가?
System.out.println("파일 있다");
long filesize=file.length();
System.out.println("파일크기 : " + filesize);
System.out.println("파일크기 : " + (filesize/1024) + "KB");
String filename=file.getName();
System.out.println("파일명 : " + filename);
//문제) 파일명과 확장명을 분리해서 출력하시오.
//파일명 : python-3.8.6
//확장명 : exe
int lastdot=filename.lastIndexOf(".");
String name=filename.substring(0, lastdot);
String ext=filename.substring(lastdot+1);
System.out.println("파일명 : " + name);
System.out.println("확장명 : " + ext);
//파일 삭제
//->실제 파일이 삭제되니 복사본 남겨 놓으시기 바랍니다
file.delete(); //파일이 사라졌어!!!
}else {
System.out.println("파일 없다");
}//if end
}catch (Exception e) {
//예외가 발생되면 처리할 코드를 작성
System.out.println("File 클래스 실패 : " + e);
}//end
System.out.println("END");
결과값
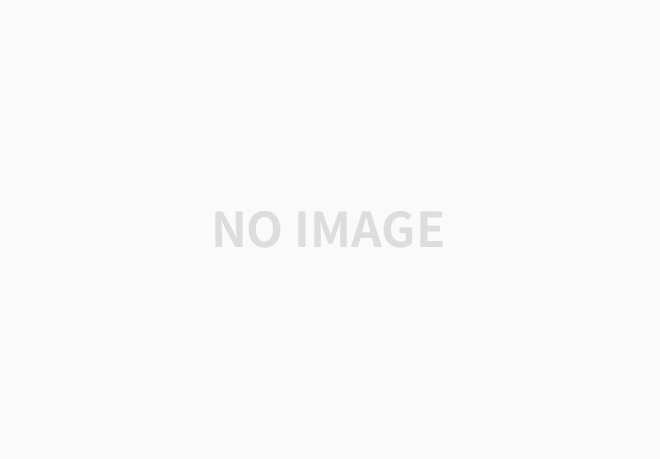
Input
- Java 기본 입출력 방식
예제1) Input
package oop0909;
import java.io.*;
public class Test05_input {
public static void main(String[] args) {
//메모장 파일 내용 읽기
FileReader fr=null;
BufferedReader br=null;
try {
String filename="C:/java0812/workspace/Java/src/oop0909/test1.txt";
//1)파일 가져오기
fr=new FileReader(filename);
//2)파일 내용 읽기(cursor가 생성됨)
br=new BufferedReader(fr);
int num=0; //행번호
while(true) {
//3)엔터(\n)를 기준으로 한 줄씩 가져오기
String line=br.readLine();
if(line==null) { //파일의 끝(End Of File)
break;
}//if end
System.out.printf("%3d %s\n", ++num, line);
//20줄마다 선 긋기
if(num%20==0) {
System.out.println("======================");
}
}//while end
} catch (Exception e) {
System.out.println("파일읽기 실패 : " + e); //java.io.FileNotFoundException
}finally {
//자원 반납 순서 주의 가장 늦게 열었던 것을 가장 먼저 닫는다
try {
if(br!=null) {br.close();}
} catch (Exception e2) {}//end
try {
if(fr!=null) {fr.close();}
} catch (Exception e2) {}//end
}//end
}//main() end
}//class end
결과값
1 hello
2 hello
...
19 hello
20 hello
======================
21 hello
22 hello
...
39 hello
40 hello
======================
예제2) InputStream 기반과 Reader 기반 비교
package oop0909;
import java.io.FileInputStream;
public class Test06_input {
public static void main(String[] args) {
//InputStream기반과 Reader기반 비교
String filename="C:/java0812/workspace/Java/src/oop0909/test2.txt";
try {
//byte형 : 1바이트 할당
//char형 : 2바이트 할당
//1)FileInputStream : 1바이트 기반
//->한글 깨짐
FileInputStream fis=new FileInputStream(filename);
while(true) {
int data=fis.read(); //1바이트 읽기
if(data==-1) { //파일의 끝(End of File)인지?
break;
}//if end
System.out.printf("%c", data);
}//while end
fis.close(); //자원 반납
} catch (Exception e) {
System.out.println("실패 : "+e);
}//end
}//main() end
}//class end
결과값
hello
ìë
hello
ìë
...
hello
ìë
hello
ìë
1바이트 단위로 읽어내려가기 때문에 2바이트 단위인 한글이 깨진다.
여기서 주의해야 할 사항은 파일의 끝(End of File)을 data==-1 형식으로 사용한다는 것이다. (★★)
package oop0909;
import java.io.FileReader;
public class Test06_input {
public static void main(String[] args) {
//InputStream기반과 Reader기반 비교
String filename="C:/java0812/workspace/Java/src/oop0909/test2.txt";
try {
//byte형 : 1바이트 할당
//char형 : 2바이트 할당
//2)FileReader : char(2바이트) 기반
//->한글 안 깨짐
FileReader fr=new FileReader(filename);
while(true) {
int data=fr.read();
if(data==-1) { //data==-1 파일의 끝
break;
}//if end
System.out.printf("%c", data);
}//while end
fr.close();
} catch (Exception e) {
System.out.println("실패 : "+e);
}//end
}//main() end
}//class end
결과값
hello
안녕
hello
안녕
...
hello
안녕
hello
안녕
reader 형식은 char 단위로 읽어내려가기 때문에 2바이트 단위인 한글은 깨지지 않는다.
Output
- Java 기본 입출력 방식
예제) 메모장 파일에 출력
package oop0909;
import java.io.FileWriter;
import java.io.PrintWriter;
import jdk.jfr.events.FileWriteEvent;
public class Test07_output {
public static void main(String[] args) {
//메모장 파일에 출력하기
String fileName="C:/java0812/00/sungjuk.txt";
//출력파일(sungjuk.txt)이
//->없으면 파일을 생성한다(create)
//->있으면 덮어쓰기(overwrite)를 할 것인지 또는 추가(append)할 것인지 선택할 수 있다
try {
//true : append된다
//false: overwrite된다
FileWriter fw=new FileWriter(fileName, false);
//autoFlush: true
//->버퍼클리어
PrintWriter out=new PrintWriter(fw, true);
out.println("무궁화,95,90,100");
out.println("홍길동,100,100,100");
out.println("라일락,90,95,35");
out.println("개나리,85,70,75");
out.println("진달래,35,40,60");
System.out.println("sungjuk.txt 데이터 파일 완성");
//자원 반납
out.close(); fw.close();
} catch (Exception e) {
System.out.println("파일쓰기 실패 : "+e);
}//end
}//main() end
}//class end
결과값
sungjuk.txt 데이터 파일 완성
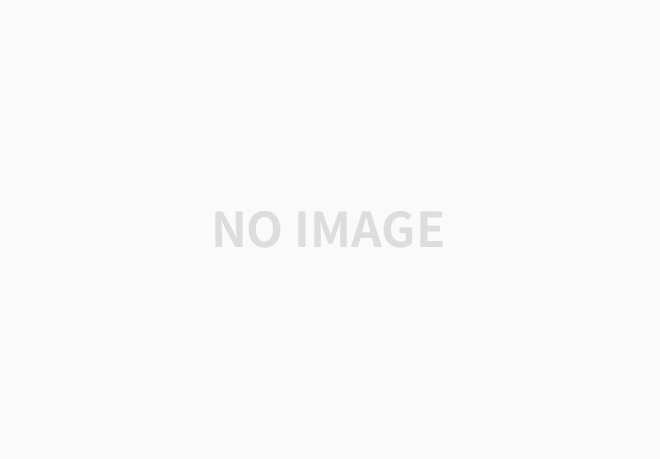
문제) 성적프로그램
package oop0909;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.PrintWriter;
import java.util.StringTokenizer;
public class Test08_sungjuk {
public static void main(String[] args) {
// 연습문제) 성적프로그램
/*
1) 입력파일: sungjuk.txt
2) 출력결과 파일 : result.txt
형식)
성 / 적 / 결 / 과
---------------------------------------------------
이름 국어 영어 수학 평균 등수 결과
---------------------------------------------------
무궁화 100 100 100 100 1 합격 ********** 장학생
홍길동 20 50 30 33 5 불합격 ***
개나리 90 95 90 91 2 합격*********
진달래 70 80 60 70 4 합격 *******
라일락 35 100 100 78 3 재시험 *******
---------------------------------------------------
*/
try {
String inName = "C:/java0812/00/sungjuk.txt";
String outName = "C:/java0812/00/result.txt";
//1)단계: 데이터 저장 변수 선언 -> 변수배열선언
String name[] = new String[5];
int kor[] = new int[5];
int eng[] = new int[5];
int mat[] = new int[5];
int avg[] = new int[5];
int rank[] = { 1, 1, 1, 1, 1 };
int size = name.length;
int i=0; //행번호
//sungjuk.txt파일 열기
FileReader fr = new FileReader(inName);
BufferedReader br = new BufferedReader(fr); //cursor
//줄 단위로 불러와서 자르기
//엔터(\n)를 기준으로 한 줄씩 가져오기
//, 기준으로 잘라서 각각의 변수배열에 입력
while(true) {
String line=br.readLine(); //"무궁화,95,90,100"
if(line==null) { //파일의 끝(End Of File)
break;
}//if end
//,를 기준으로 문자열 분리한 후 1)단계에서 선언한 변수에 저장하기
//1)StringTokenizer 활용
StringTokenizer st=new StringTokenizer(line, ",");
while(st.hasMoreElements()) {
/*
int countTokens = st.countTokens();
for(i=0; i<countTokens; i++) {
name[i]=st.nextToken();
kor[i]=Integer.parseInt(st.nextToken());
eng[i]=Integer.parseInt(st.nextToken());
mat[i]=Integer.parseInt(st.nextToken());
}//for end
*/
//요소값
name[i]=st.nextToken();
kor[i]=Integer.parseInt(st.nextToken()); //자바형변환
eng[i]=Integer.parseInt(st.nextToken());
mat[i]=Integer.parseInt(st.nextToken());
}//while end(배열의 입력 자료 완료)
//2)split()함수 활용 (숙제)
//split
i++; //다음 사람
}//while end
//3)단계: 평균 구하기
for(i=0; i<size; i++) {
avg[i]=(kor[i]+eng[i]+mat[i])/3;
}//for end
//4)단계: 등수 구하기
for(int a=0; a<size; a++) {
for(int b=0; b<size; b++) {
if(avg[a]<avg[b]) {
rank[a]=rank[a]+1;
}//if end
}//for end
}//for end
//5)단계: result.txt 출력하기
FileWriter fw=new FileWriter(outName, false);
//autoFlush : true 버퍼 클리어
PrintWriter out=new PrintWriter(fw, false);
out.println(" 성 / 적 / 결 / 과 ");
out.println("-------------------------------------------------------------------");
out.println("이름 국어 영어 수학 평균 등수 결과 ");
out.println("-------------------------------------------------------------------");
//순서대로 출력
for(i=0; i<size; i++) {
out.printf("%-3s %6d %6d %6d %6d %3d "
,name[i],kor[i],eng[i],mat[i],avg[i],rank[i]);
//합격, 불합격 여부
if(avg[i]>=70) {
if(kor[i]<40 || eng[i]<40 || mat[i]<40) {
out.printf("%-5s", "재시험 ");
}else {
out.printf("%-5s", "합 격 ");
}//if end
}else {
out.printf("%-5s", "불합격 ");
}//if end
//별*
for(int star=1; star<=avg[i]/10; star++) {
out.printf("%c", '*');
}//for end
//평균 95점 이상이면 장학생
if(avg[i]>=95) {
out.printf("%-12s", "장학생");
}//if end
//다음줄로 가자
out.println(); //한 사람 출력하면 줄 바꿈
}//for end
//자원 반납
br.close(); fr.close(); //버전 영향을 받는 것 같다
out.close(); fw.close();
} catch (Exception e) {
System.out.println("성적프로그램 실패:" + e);
} // end
System.out.println("END");
}// main() end
}// class end
결과값
성 / 적 / 결 / 과
---------------------------------------------------------------------------
이름 국어 영어 수학 평균 등수 결과
---------------------------------------------------------------------------
무궁화 95 90 100 95 2 합 격 *********장학생
홍길동 100 100 100 100 1 합 격 **********장학생
라일락 90 95 35 73 4 재시험 *******
개나리 85 70 75 76 3 합 격 *******
진달래 35 40 60 45 5 불합격 ****
'자바 웹개발자 과정 > JAVA' 카테고리의 다른 글
200910 OOP 15 - Java 객체지향 프로그래밍 (Ⅱ) (0) | 2020.09.10 |
---|---|
200908 OOP 13 - Java 객체지향 프로그래밍 (Ⅱ) (0) | 2020.09.09 |
200831 ~ 0904, 0907 - 코로나 2.5단계에 의한 휴강 (0) | 2020.09.07 |
200828 OOP 12 - Java 객체지향 프로그래밍 (Ⅱ) (0) | 2020.09.07 |
200827 OOP 11 - Java 객체지향 프로그래밍(Ⅰ, Ⅱ) (0) | 2020.08.27 |